João Pedro Cosso - BlogHow to Prepare for a Technical Interview in Software Development
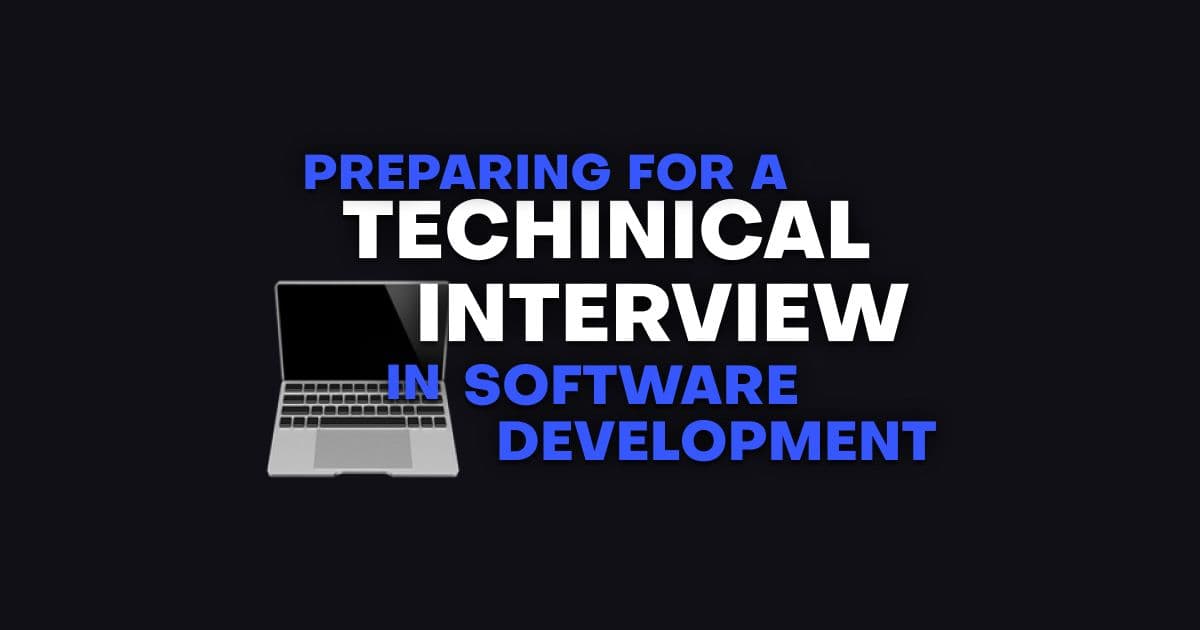
1. Understand the Interview Format
Technical interviews share some common components:
- Coding Challenges: Solve algorithmic or coding problems, often under time constraints.
- System Design: Design scalable systems or discuss architectural decisions (for mid to senior levels).
- Behavioral Questions: Assess problem-solving approaches, teamwork, and how you handle challenges.
For all levels, understanding the interview structure is crucial. Start by researching the company’s specific interview style. Larger tech firms (e.g., Google, Amazon) focus on algorithms and data structures, while startups might focus more on practical problem-solving and your ability to adapt.
2. Sharpen Your Problem-Solving Skills
Coding challenges are a key component of most technical interviews. Websites like LeetCode, HackerRank, Codeforces, and Exercism are widely used by interviewers and candidates alike for preparation. Here’s how each level should approach coding practice:
- LeetCode: Ideal for algorithmic challenges and data structures, LeetCode has problems sorted by difficulty and covers a wide range of topics asked in interviews. Start with easy problems and work your way up to harder ones.
- HackerRank: Offers a diverse set of challenges from basic to advanced, along with interview preparation kits. It’s great for improving general problem-solving skills and coding fundamentals.
- Codeforces: Known for its competitive programming contests, Codeforces is great for those who want to practice under pressure. It also includes timed problem-solving which is beneficial for interviews.
For Junior Developers:
Junior candidates often need to focus on mastering the fundamentals of coding and algorithms. Here’s what to focus on:
- Data Structures & Algorithms: Focus on mastering basic data structures like arrays, linked lists, stacks, queues, hash maps, and binary trees. For algorithms, work on sorting, searching, and basic recursion problems.
- Platform Practice: Start with LeetCode Easy or HackerRank Easy/Medium problems. Focus on solving problems from categories like string manipulation, basic math, and fundamental data structures.
- Key Soft Skills: Be prepared for behavioral questions that assess your problem-solving mindset and ability to learn. Since you may not have extensive experience, demonstrating enthusiasm, quick learning abilities, and a strong foundation is critical.
For Mid-Level Developers:
Mid-level developers are expected to solve more complex problems and may need to start thinking about system design questions:
- Advanced Algorithms & Data Structures: Along with the basics, focus on more advanced topics like dynamic programming, graph theory, and tree traversal algorithms. You should also be comfortable with understanding time and space complexity.
- System Design (Introductory): Start practicing small-scale system design problems. You won’t be expected to design massive systems but should have a grasp of components like caching, database indexing, and simple load balancing.
- Platform Practice: Work on LeetCode Medium and HackerRank Medium/Hard problems. Sites like Exercism are great for improving your code quality and problem-solving approach.
- Key Soft Skills: At this stage, focus on communication and teamwork skills. Be ready to discuss how you’ve solved technical challenges in past projects and how you contribute to the success of a team.
For Senior Developers:
Senior-level candidates are expected to handle complex system design, optimization problems, and demonstrate leadership in technical problem-solving:
- System Design: For senior roles, system design becomes a critical focus. Be familiar with architectural concepts like microservices, load balancing, sharding, replication, and fault tolerance. Platforms like Grokking the System Design Interview and Educative.io offer deep dives into common system design patterns.
- Optimization: For coding challenges, expect questions around optimization and scalability. You’ll be asked to write not just correct code, but also optimized solutions that can handle large datasets or high traffic.
- Platform Practice: Focus on LeetCode Hard problems and systems design challenges on InterviewBit or Pramp. Senior roles may also involve whiteboarding sessions where you must articulate complex architectural solutions.
- Key Soft Skills: Leadership and decision-making are crucial. Be prepared to explain how you’ve led projects, made architectural decisions, and mentored junior developers.
3. Master System Design (For Mid to Senior Levels)
For mid and senior-level roles, system design interviews play a significant role. You might be asked to design large-scale systems like social networks, content distribution platforms, or even payment systems. Here’s what to focus on:
- Scalability & Reliability: Understand core concepts like horizontal scaling, partitioning, and redundancy. For instance, you may need to design a system that can handle millions of users while remaining highly available.
- APIs and Microservices: Know how to design RESTful APIs and microservice architectures that can scale and be maintained independently.
- Cloud & Distributed Systems: Be aware of cloud infrastructure (AWS, Azure, GCP) and distributed system patterns like message queues, eventual consistency, and distributed data storage.
- Resources: Use Grokking the System Design Interview and System Design Primer (open-source on GitHub) to familiarize yourself with common system design interview questions.
4. Strengthen Your Core Programming Skills
Regardless of experience level, you must be proficient in at least one programming language. Here’s how each level can approach core programming skills:
- Junior Developers: Focus on becoming fluent in one language (e.g., Python, JavaScript, Java, C++). You don’t need to be an expert in multiple languages, but you should know one inside out, including common libraries, syntax, and idiomatic coding practices.
- Mid-Level Developers: Build on your foundational skills by learning best practices for writing clean, maintainable code. This includes adhering to SOLID principles, improving code modularity, and understanding testing frameworks.
- Senior Developers: Senior developers need to demonstrate a deep understanding of code optimization, profiling, and scaling. You should be able to optimize for performance (time and space complexity) and write highly maintainable, production-ready code. Knowledge of advanced debugging techniques and code refactoring is also key.
5. Prepare for Behavioral Questions
Behavioral interviews are a crucial part of the process, and expectations differ by experience level.
- Junior Developers: Expect questions like “Tell me about a time you faced a challenge while learning something new.” Employers are looking for adaptability and willingness to grow.
- Mid-Level Developers: You’ll need to discuss past projects where you’ve worked in teams, handled conflicts, or solved complex technical issues. Be ready to demonstrate ownership of tasks and collaborative problem-solving.
- Senior Developers: Senior candidates should be prepared to talk about leadership, mentorship, and making high-level decisions. Questions like “Tell me about a time you made a critical architectural decision” or “How do you handle conflicting opinions in a technical discussion?” are common.
Use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
6. Simulate the Interview Environment
Regardless of your level, practice mock interviews in real-time scenarios. Platforms like Pramp, Interviewing.io, and Exercism allow you to practice live coding with a peer or professional. These platforms simulate the pressure of real interviews and provide valuable feedback on both your technical and communication skills.
7. Know the Company and Role
Tailor your preparation to the specific company. For example, companies like Amazon may have leadership principles that are deeply embedded into their interviews, while smaller companies might prioritize a cultural fit and practical problem-solving. Focus on:
- Technical Stack: Research the company’s technical stack to understand their tools, languages, and frameworks. This helps align your answers and demonstrate your ability to fit in.
- Company Products: Have a solid understanding of the company’s products, challenges, and mission. This shows genuine interest and makes your answers more relevant.
8. Final Tips for Interview Day
- Stay Calm and Communicate: Talk through your solutions clearly. If you're stuck, ask clarifying questions and approach the problem step-by-step.
- Ask for Feedback: If you're unsure about your solution, ask the interviewer for guidance. This shows that you're open to feedback and can adjust your approach as needed.
- Follow-Up: Send a thank-you note after your interview. Mention something specific from the conversation to leave a lasting positive impression.
Conclusion 🏁
Preparing for a technical interview in software development requires focus, discipline, and practice. Whether you're just starting out or applying for a senior role, it’s important to sharpen your technical skills, practice real-world problem-solving, and prepare for behavioral questions. By leveraging the right resources like LeetCode, HackerRank, Exercism, and *Grokking the System